Introduction
In the fast-paced world of software development, writing clean, scalable, and maintainable code is a challenge that developers face daily. Without proper structure, applications can quickly become tangled, leading to bugs, inefficiencies, and increased maintenance costs. This is where the SOLID principles of Object-Oriented Design come into play.
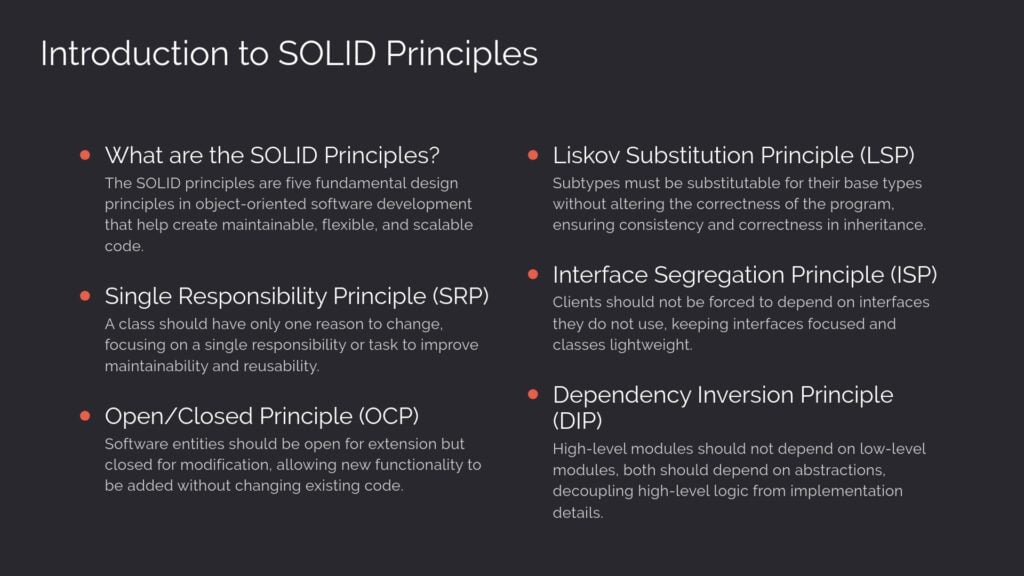
First introduced by Robert C. Martin (Uncle Bob), SOLID is an acronym representing five fundamental principles that guide developers in creating robust, modular, and adaptable software. These principles ensure that code remains flexible to change, easy to understand, and resilient to future requirements.
By mastering Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion principles, developers can design systems that are not only easier to extend but also more resistant to breaking when modified.
In this article, we’ll explore each of the SOLID principles in depth, providing clear definitions, real-world examples, and best practices to help you apply them effectively.
Here’s an improved outline for your article, including a Table of Contents to enhance readability and navigation:
Table of Contents
- Introduction
- Understanding SOLID Principles
- Single Responsibility Principle (SRP)
- Open/Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
- Benefits of Applying SOLID Principles
- Common Mistakes and How to Avoid Them
- Conclusion
Understanding SOLID Principles
The SOLID principles serve as a foundation for writing high-quality, maintainable, and scalable object-oriented software. Each principle addresses a common design challenge, helping developers build systems that are modular, adaptable, and easier to debug.
When applied correctly, these principles lead to:
✅ Better Code Maintainability – Code is easier to read, modify, and extend.
✅ Reduced Technical Debt – Avoids messy, tightly coupled code that becomes difficult to manage.
✅ Improved Testability – Smaller, well-defined components make unit testing more effective.
✅ Enhanced Collaboration – Clean, modular design simplifies teamwork and onboarding.
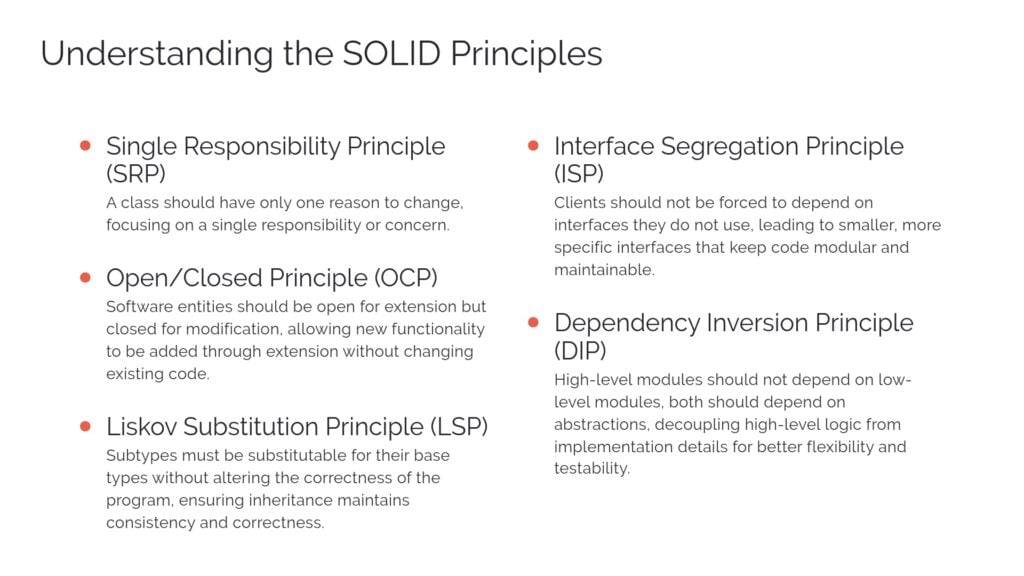
Breaking Down the SOLID Acronym
SOLID is an acronym for five key principles of object-oriented design:
- Single Responsibility Principle (SRP) – A class should have only one reason to change.
- Open/Closed Principle (OCP) – Software entities should be open for extension but closed for modification.
- Liskov Substitution Principle (LSP) – Subtypes should be replaceable for their base types without altering behavior.
- Interface Segregation Principle (ISP) – Clients should not be forced to depend on interfaces they do not use.
- Dependency Inversion Principle (DIP) – High-level modules should not depend on low-level modules. Both should depend on abstractions.
Each of these principles reduces complexity, minimizes dependencies, and promotes better software design. In the following sections, we’ll take a closer look at each principle, exploring practical examples and best practices for implementation.
Single Responsibility Principle (SRP)
Definition
The Single Responsibility Principle (SRP) states that a class should have only one reason to change. A class should focus on one responsibility rather than handling multiple concerns.
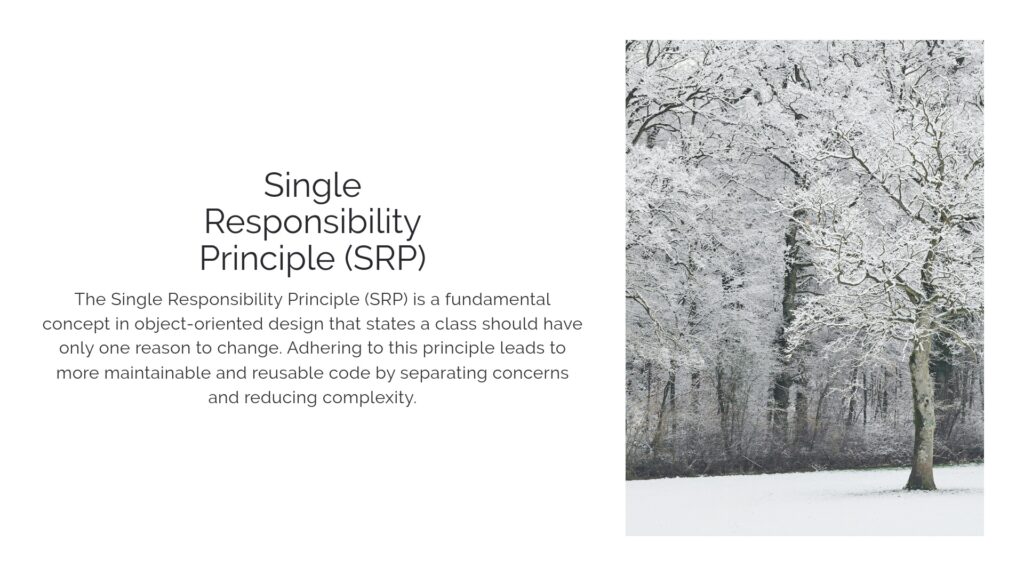
Why SRP Matters
Violating SRP can lead to:
❌ Increased complexity – Changes to one functionality may affect another.
❌ Harder debugging – A single class handling multiple concerns makes testing difficult.
❌ Poor reusability – Mixing multiple concerns makes it hard to reuse classes elsewhere.
By following SRP, we create smaller, more maintainable, and reusable classes.
Example of SRP Violation
Consider a class that manages user data and also generates reports:
pythonCopyEditclass User:
def __init__(self, name, email):
self.name = name
self.email = email
def save_to_database(self):
print(f"Saving {self.name} to database")
def generate_report(self):
print(f"Generating report for {self.name}")
🔴 What’s Wrong?
This class has two responsibilities:
- Saving user data (database interaction).
- Generating reports (business logic for reports).
If we need to change the way reports are generated, we risk modifying user-related logic, breaking SRP.
Refactoring to Follow SRP
To comply with SRP, let’s separate the responsibilities into different classes:
pythonCopyEditclass User:
def __init__(self, name, email):
self.name = name
self.email = email
class UserRepository:
def save(self, user: User):
print(f"Saving {user.name} to database")
class UserReportGenerator:
def generate_report(self, user: User):
print(f"Generating report for {user.name}")
✅ Now, each class has a single responsibility:
User
→ Represents a user.UserRepository
→ Handles database operations.UserReportGenerator
→ Manages report generation.
This makes our code easier to test, modify, and extend.
Best Practices for Applying SRP in Python
✔ Separate concerns – Keep classes focused on one responsibility.
✔ Use composition – Break functionality into separate, dedicated classes.
✔ Follow the “one reason to change” rule – A class should only change for one specific reason.
By following SRP, we build modular, scalable, and maintainable applications. Up next, we’ll explore the Open/Closed Principle (OCP) and how it helps extend functionality without modifying existing code.
Open/Closed Principle (OCP)
Definition
The Open/Closed Principle (OCP) states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification.
This means that we should be able to add new functionality without changing existing code, which helps prevent introducing new bugs in stable code.
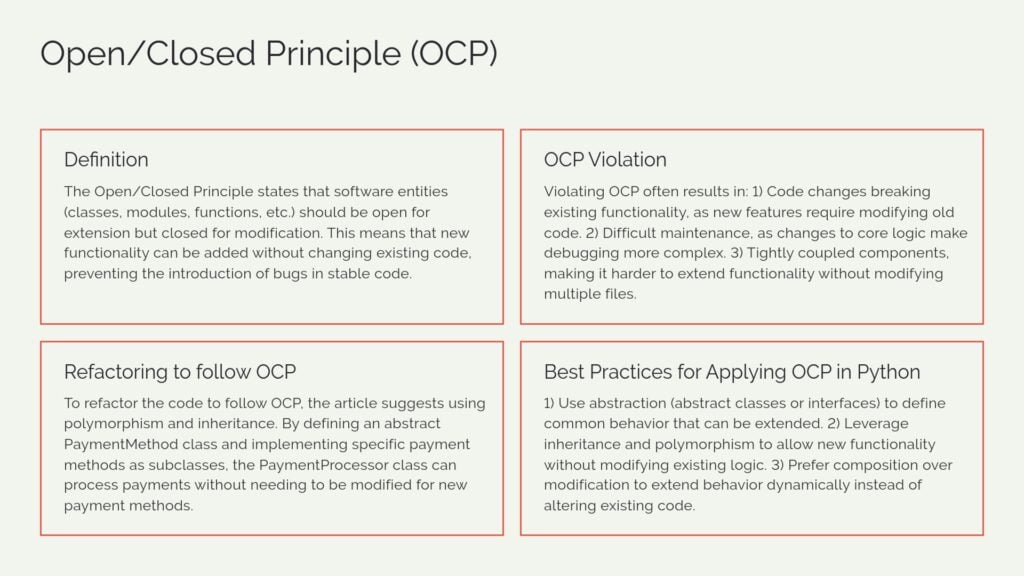
Why OCP Matters
Violating OCP often results in:
❌ Code changes breaking existing functionality – Every time a new feature is added, old code must be modified.
❌ Difficult maintenance – Changes to core logic make debugging more complex.
❌ Tightly coupled components – Harder to extend functionality without modifying multiple files.
By following OCP, we make our code flexible, scalable, and easier to maintain.
Example of OCP Violation
Let’s say we have a payment processing system that handles different payment methods:
pythonCopyEditclass PaymentProcessor:
def process_payment(self, payment_type):
if payment_type == "credit_card":
print("Processing credit card payment")
elif payment_type == "paypal":
print("Processing PayPal payment")
else:
print("Unsupported payment method")
🔴 What’s Wrong?
- Every time we add a new payment method, we must modify the
process_payment
method. - This violates OCP because the class is not closed for modification—we keep changing it for new functionality.
Refactoring to Follow OCP
A better approach is to use polymorphism and inheritance to extend functionality without modifying existing code:
pythonCopyEditfrom abc import ABC, abstractmethod
# Define an abstract class for all payment methods
class PaymentMethod(ABC):
@abstractmethod
def process_payment(self):
pass
# Implement specific payment methods
class CreditCardPayment(PaymentMethod):
def process_payment(self):
print("Processing credit card payment")
class PayPalPayment(PaymentMethod):
def process_payment(self):
print("Processing PayPal payment")
# The PaymentProcessor class no longer needs modification when adding new payment methods
class PaymentProcessor:
def process(self, payment: PaymentMethod):
payment.process_payment()
✅ Now, when we need a new payment method, we extend instead of modifying existing code:
pythonCopyEditclass BitcoinPayment(PaymentMethod):
def process_payment(self):
print("Processing Bitcoin payment")
# Using the OCP-compliant design
processor = PaymentProcessor()
processor.process(CreditCardPayment()) # Output: Processing credit card payment
processor.process(PayPalPayment()) # Output: Processing PayPal payment
processor.process(BitcoinPayment()) # Output: Processing Bitcoin payment
Best Practices for Applying OCP in Python
✔ Use abstraction (abstract classes or interfaces) – Define common behavior that can be extended.
✔ Leverage inheritance and polymorphism – Allow new functionality without modifying existing logic.
✔ Prefer composition over modification – Extend behavior dynamically instead of altering existing code.
By following OCP, we ensure that our system is flexible and scalable, allowing new features to be added seamlessly.
Next, we’ll explore the Liskov Substitution Principle (LSP) and how it ensures proper inheritance design without breaking functionality.
Liskov Substitution Principle (LSP)
Definition
The Liskov Substitution Principle (LSP) states that subtypes must be substitutable for their base types without altering the correctness of the program.
This means that if a class inherits from another class, it should behave in a way that doesn’t break the expectations set by the parent class.
If a subclass modifies behavior in a way that makes the base class unusable or leads to unexpected results, it violates LSP.
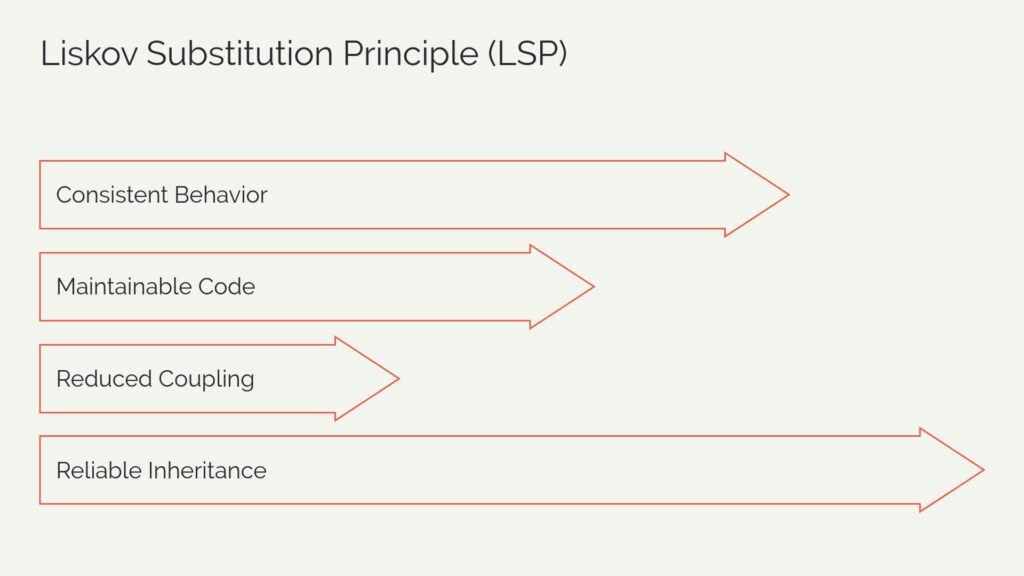
Why LSP Matters
Violating LSP leads to:
❌ Unexpected behavior – If a subclass overrides behavior incorrectly, the program might break.
❌ Tight coupling – Code dependent on a base class might fail when given a subclass.
❌ Difficult debugging – Inconsistencies in behavior lead to hidden bugs.
By following LSP, we ensure that inheritance maintains consistency and correctness.
Example of LSP Violation
Let’s say we have a base class Bird
, and a subclass Penguin
.
pythonCopyEditclass Bird:
def fly(self):
print("Flying high!")
class Penguin(Bird):
def fly(self):
raise Exception("Penguins cannot fly!")
🔴 What’s Wrong?
- A Penguin is a Bird, but it breaks the
fly()
method. - If a function expects a
Bird
, it might crash if it gets aPenguin
.
pythonCopyEditdef make_bird_fly(bird: Bird):
bird.fly() # This will throw an exception if bird is a Penguin
make_bird_fly(Penguin()) # ❌ Exception: Penguins cannot fly!
Since Penguin
violates the behavior of Bird
, it violates LSP.
Refactoring to Follow LSP
Instead of forcing all birds to have fly()
, we should separate flying and non-flying birds.
pythonCopyEditfrom abc import ABC, abstractmethod
# Base class for all birds
class Bird(ABC):
def __init__(self, name):
self.name = name
@abstractmethod
def move(self):
pass
# Subclass for birds that can fly
class FlyingBird(Bird):
def move(self):
print(f"{self.name} is flying!")
# Subclass for birds that cannot fly
class NonFlyingBird(Bird):
def move(self):
print(f"{self.name} is walking!")
# Now, Penguin does not break expectations
class Penguin(NonFlyingBird):
pass
# Usage
def make_bird_move(bird: Bird):
bird.move()
make_bird_move(FlyingBird("Sparrow")) # ✅ Output: Sparrow is flying!
make_bird_move(Penguin("Penguin")) # ✅ Output: Penguin is walking!
✅ Now, Penguin does not override fly()
incorrectly, and the Bird
class is properly structured.
Best Practices for Applying LSP in Python
✔ Check if subclasses can replace base classes without breaking behavior.
✔ Use abstract base classes (ABC) to define expected behavior clearly.
✔ Avoid forcing behaviors that don’t apply to all subclasses (e.g., making all birds fly).
By following LSP, we ensure consistency in our object hierarchy and avoid unexpected bugs.
I found this meme on the internet a while ago, but I can’t remember where. I wish I could give attribution to it. But I think it helps describe the Liskov substitution Principle very well:
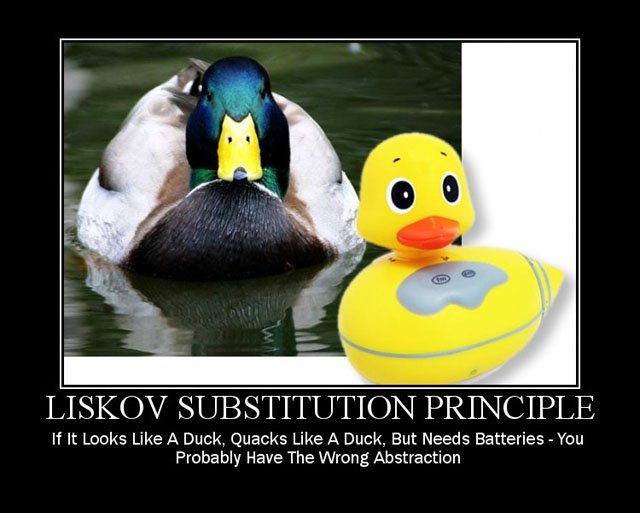
In this meme, we might have a duck class. But only one of them takes batteries. If the real duck is a subclass of the rubber duck, then we have a problem because the regular duck does not use batteries.
Next, we’ll explore the Interface Segregation Principle (ISP) and why splitting large interfaces makes code more modular and maintainable.
Interface Segregation Principle (ISP)
Definition
The Interface Segregation Principle (ISP) states that clients should not be forced to depend on interfaces they do not use.
In simpler terms, a class should not be required to implement methods that it doesn’t need. Instead of large, bloated interfaces, we should create smaller, more specific ones to keep our code modular and maintainable.
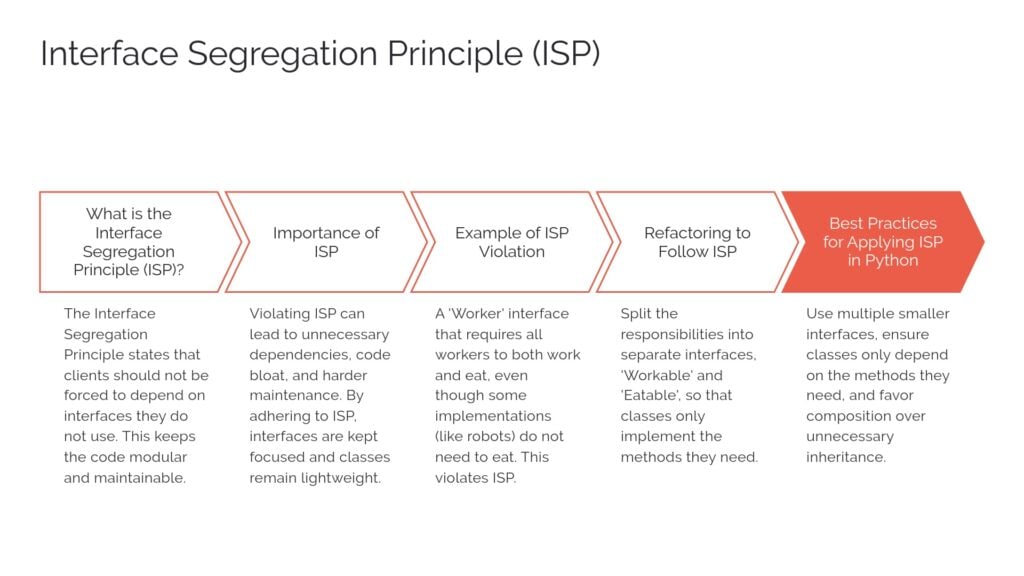
Why ISP Matters
Violating ISP often results in:
❌ Unnecessary dependencies – Classes may be forced to implement irrelevant methods.
❌ Code bloat – Large interfaces make code more difficult to understand and modify.
❌ Harder maintenance – If an interface changes, all implementing classes must be updated, even if they don’t use the modified method.
By following ISP, we keep interfaces focused and classes lightweight.
Example of ISP Violation
Imagine we have a Worker
interface that requires all workers to both work and eat:
pythonCopyEditfrom abc import ABC, abstractmethod
class Worker(ABC):
@abstractmethod
def work(self):
pass
@abstractmethod
def eat(self):
pass
class HumanWorker(Worker):
def work(self):
print("Human is working")
def eat(self):
print("Human is eating")
class RobotWorker(Worker):
def work(self):
print("Robot is working")
def eat(self):
raise Exception("Robots do not eat!") # ❌ Violates ISP
🔴 What’s Wrong?
- The
RobotWorker
is forced to implementeat()
, even though robots do not eat. - This violates ISP because
Worker
contains methods that not all implementations need.
Refactoring to Follow ISP
Instead of one large Worker
interface, we split responsibilities into separate interfaces:
pythonCopyEditclass Workable(ABC):
@abstractmethod
def work(self):
pass
class Eatable(ABC):
@abstractmethod
def eat(self):
pass
class HumanWorker(Workable, Eatable):
def work(self):
print("Human is working")
def eat(self):
print("Human is eating")
class RobotWorker(Workable):
def work(self):
print("Robot is working")
# Now, RobotWorker does not need to implement eat()
✅ Now, RobotWorker
only implements the necessary interface (Workable
), and HumanWorker
implements both Workable
and Eatable
.
Best Practices for Applying ISP in Python
✔ Use multiple smaller interfaces instead of one large one.
✔ Ensure that classes only depend on the methods they need.
✔ Favor composition over unnecessary inheritance to keep responsibilities separate.
By following ISP, we create modular, reusable, and easy-to-maintain code.
Next, we’ll explore the Dependency Inversion Principle (DIP) and how it helps decouple high-level and low-level modules for better flexibility.
Dependency Inversion Principle (DIP)
Definition
The Dependency Inversion Principle (DIP) states that high-level modules should not depend on low-level modules. Both should depend on abstractions.
This means that instead of hardcoding dependencies between classes, we should rely on interfaces (abstractions) to make our code more flexible and maintainable.
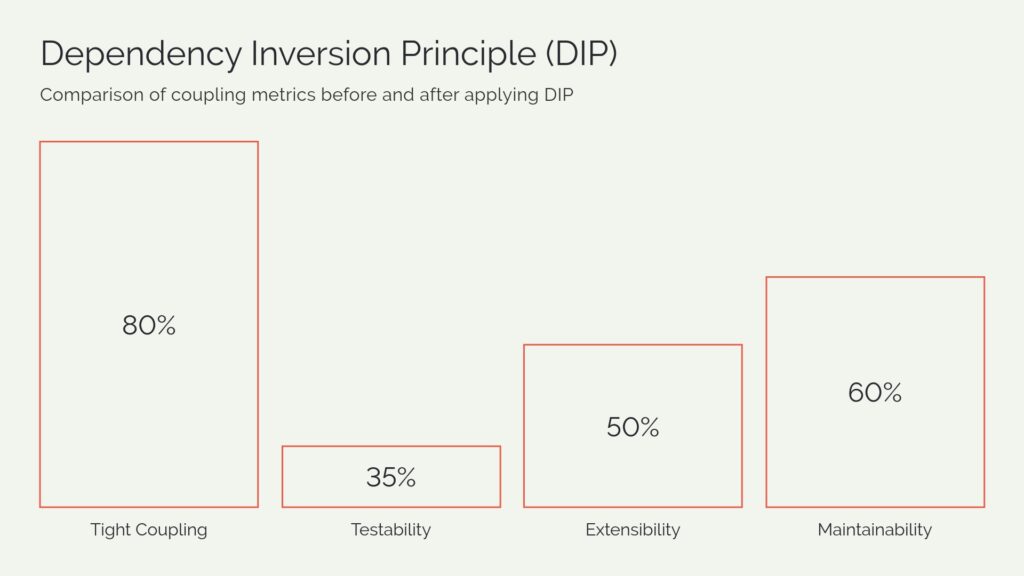
Why DIP Matters
Violating DIP leads to:
❌ Tightly coupled code – Changes in low-level modules can break high-level modules.
❌ Difficult testing – Hardcoded dependencies make unit testing harder.
❌ Poor flexibility – Extending functionality requires modifying existing code.
By following DIP, we decouple high-level logic from implementation details, making it easier to change and test.
Example of DIP Violation
Let’s say we have a Keyboard
class that our Computer
class depends on directly:
pythonCopyEditclass Keyboard:
def connect(self):
return "Keyboard connected"
class Computer:
def __init__(self):
self.keyboard = Keyboard() # ❌ Hardcoded dependency
def use(self):
print(self.keyboard.connect())
🔴 What’s Wrong?
Computer
is tightly coupled toKeyboard
.- If we want to use a different input device (e.g., Mouse, Touchscreen), we must modify
Computer
.
Refactoring to Follow DIP
Instead of directly depending on Keyboard
, we define an abstraction (InputDevice
) and depend on that instead:
pythonCopyEditfrom abc import ABC, abstractmethod
# Define an abstraction for input devices
class InputDevice(ABC):
@abstractmethod
def connect(self):
pass
# Implement specific input devices
class Keyboard(InputDevice):
def connect(self):
return "Keyboard connected"
class Mouse(InputDevice):
def connect(self):
return "Mouse connected"
# Computer depends on the abstraction, not a specific device
class Computer:
def __init__(self, input_device: InputDevice):
self.input_device = input_device # ✅ Dependency Injection
def use(self):
print(self.input_device.connect())
# Usage
computer1 = Computer(Keyboard())
computer1.use() # ✅ Output: Keyboard connected
computer2 = Computer(Mouse())
computer2.use() # ✅ Output: Mouse connected
✅ Now, Computer
does not depend on a specific device—it depends on an abstraction (InputDevice
), making it flexible and extensible.
Best Practices for Applying DIP in Python
✔ Depend on abstractions, not concrete classes.
✔ Use dependency injection to pass dependencies into a class instead of hardcoding them.
✔ Favor composition over inheritance to make components interchangeable.
By following DIP, we create flexible, testable, and easily extendable software.
This wraps up the SOLID principles! In the next section, we’ll discuss the benefits of applying SOLID principles and how they improve software design.
Also See: Types of software testing!
Benefits of Applying SOLID Principles
Applying the SOLID principles leads to better software design that is scalable, maintainable, and easier to test. Let’s explore some of the key benefits.
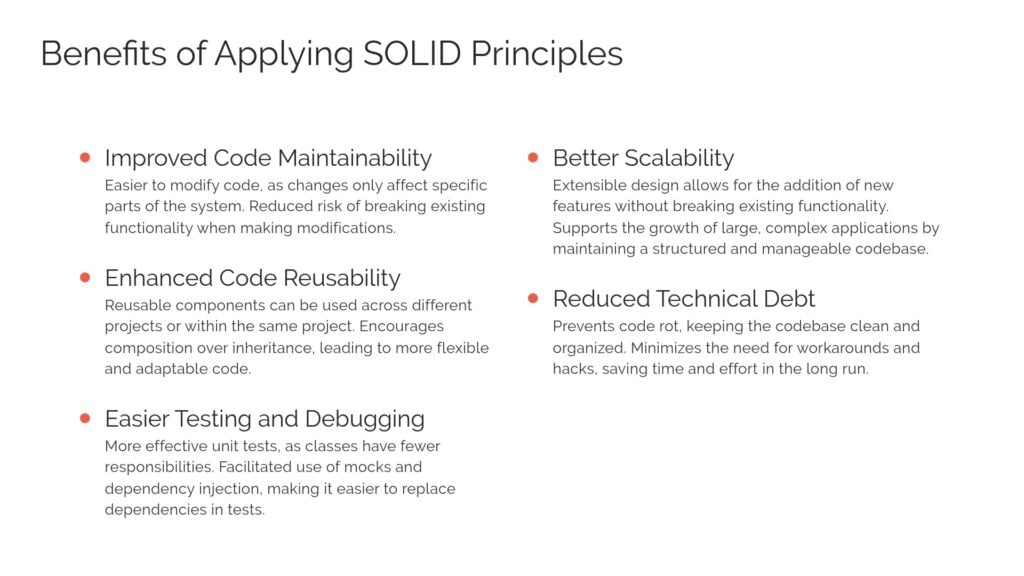
1️⃣ Improved Code Maintainability
✅ Easier to modify – Since each class has a clear responsibility, changes affect only specific parts of the code.
✅ Less risk of breaking functionality – Modifications in one part don’t cause unexpected side effects in other areas.
💡 Example:
- Following SRP (Single Responsibility Principle) ensures that modifying report generation doesn’t accidentally affect database operations.
2️⃣ Enhanced Code Reusability
✅ Reusable components – Small, modular classes can be used across different projects or in multiple places within the same project.
✅ Encourages composition over inheritance – Avoids rigid class hierarchies that make reuse difficult.
💡 Example:
- OCP (Open/Closed Principle) allows adding new payment methods to an e-commerce platform without modifying existing code.
3️⃣ Easier Testing and Debugging
✅ Unit tests are more effective – Since classes have fewer responsibilities, it’s easier to test individual components.
✅ Mocks and dependency injection – DIP (Dependency Inversion Principle) makes it easier to replace dependencies in tests.
💡 Example:
- By following DIP, we can swap out a real database connection for a mock database in unit tests, making testing faster and more reliable.
4️⃣ Better Scalability
✅ Extensible design – New features can be added easily without breaking existing functionality.
✅ Supports growing projects – Large applications remain structured and manageable over time.
💡 Example:
- ISP (Interface Segregation Principle) ensures that each class only implements the methods it actually needs, making the system more adaptable to new requirements.
5️⃣ Reduced Technical Debt
✅ Prevents code rot – Code stays clean and organized, reducing the need for future refactoring.
✅ Minimizes workarounds – Developers don’t have to hack around poor design choices, saving time and effort.
💡 Example:
- LSP (Liskov Substitution Principle) ensures that subclasses behave correctly, preventing unpredictable behavior when new features are introduced.
By applying SOLID principles, developers create high-quality, scalable, and maintainable software. These principles are not just theoretical concepts—they solve real-world software challenges and ensure that systems remain flexible and robust over time.
Up next, we’ll explore common mistakes developers make when applying SOLID and how to avoid them.
Common Mistakes and How to Avoid Them
Even though the SOLID principles help create well-structured software, they can be misunderstood or misapplied, leading to unintended consequences. Below are some common mistakes developers make when implementing SOLID principles and how to avoid them.
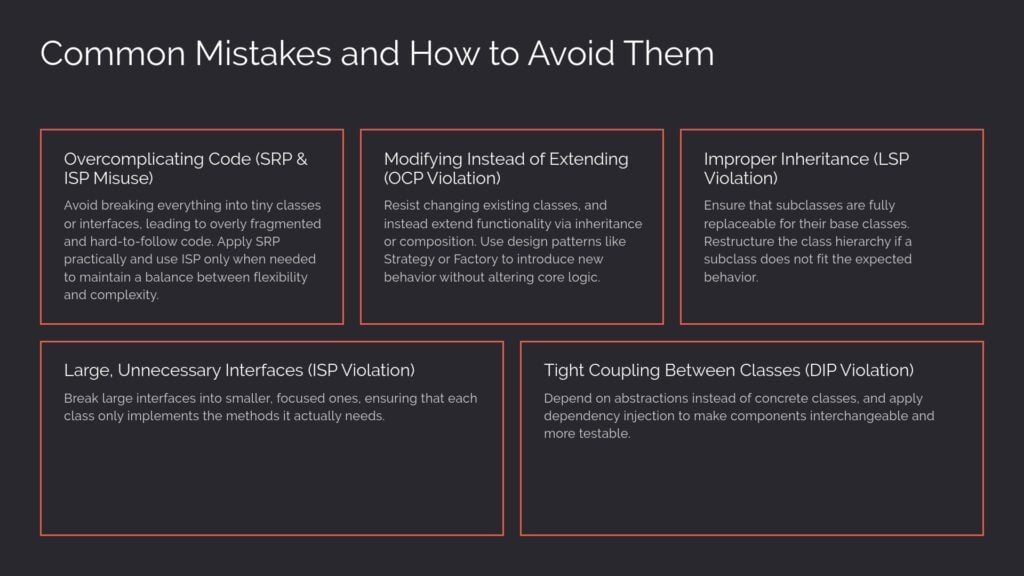
1️⃣ Overcomplicating Code (SRP & ISP Misuse)
❌ The Mistake: Trying to break everything into tiny classes or interfaces, making the code overly fragmented and hard to follow.
❌ Example: Creating too many micro-interfaces that require complex interactions, leading to confusing and inefficient code.
✅ The Solution:
✔ Apply SRP (Single Responsibility Principle) practically—not every function needs its own class.
✔ ISP (Interface Segregation Principle) should be used only when needed to avoid bloated interfaces.
✔ Aim for balance—split responsibilities only when they clearly differ.
2️⃣ Modifying Instead of Extending (OCP Violation)
❌ The Mistake: Changing existing classes instead of extending them, leading to fragile code.
❌ Example: A developer modifies a base class to add new behavior, breaking existing implementations.
✅ The Solution:
✔ Use OCP (Open/Closed Principle) to extend functionality via inheritance or composition instead of modifying existing classes.
✔ Use design patterns like Strategy or Factory to introduce new behavior without altering core logic.
3️⃣ Improper Inheritance (LSP Violation)
❌ The Mistake: Creating subclasses that break the expectations of the base class.
❌ Example: A Penguin
class inheriting from Bird
, but overriding fly()
to raise an error.
✅ The Solution:
✔ Apply LSP (Liskov Substitution Principle) correctly—subclasses should be fully replaceable for their base class.
✔ If a subclass doesn’t fit, restructure the class hierarchy (e.g., create separate FlyingBird
and NonFlyingBird
classes).
4️⃣ Large, Unnecessary Interfaces (ISP Violation)
❌ The Mistake: Creating huge interfaces that force classes to implement unused methods.
❌ Example: A Worker
interface requiring a Robot to implement an eat()
method.
✅ The Solution:
✔ Apply ISP (Interface Segregation Principle) by breaking large interfaces into smaller, focused ones.
✔ Each class should only implement the methods it actually needs.
5️⃣ Tight Coupling Between Classes (DIP Violation)
❌ The Mistake: High-level classes depending directly on low-level implementations, making changes difficult.
❌ Example: A Computer
class directly depending on a Keyboard
class, preventing other input devices from being used.
✅ The Solution:
✔ Use DIP (Dependency Inversion Principle) by depending on abstractions instead of concrete classes.
✔ Apply dependency injection to make components interchangeable and more testable.
Understanding SOLID principles is just the first step—applying them effectively requires experience and judgment. Avoiding these common mistakes ensures that your code remains scalable, flexible, and maintainable.
Next, we’ll wrap up with a final conclusion on how SOLID principles improve software design and why every developer should master them.
Conclusion
The SOLID principles are fundamental to writing clean, maintainable, and scalable object-oriented code. By following these principles, developers can create flexible architectures that accommodate change without causing unnecessary complexity or technical debt.
Key Takeaways
✅ Single Responsibility Principle (SRP): Keep classes focused on a single responsibility to improve maintainability.
✅ Open/Closed Principle (OCP): Allow classes to be extended without modifying their existing code.
✅ Liskov Substitution Principle (LSP): Ensure that subclasses can be substituted for their base classes without breaking functionality.
✅ Interface Segregation Principle (ISP): Avoid large interfaces—create smaller, specific ones tailored to the needs of different classes.
✅ Dependency Inversion Principle (DIP): Depend on abstractions, not concrete implementations, to make systems more flexible and testable.
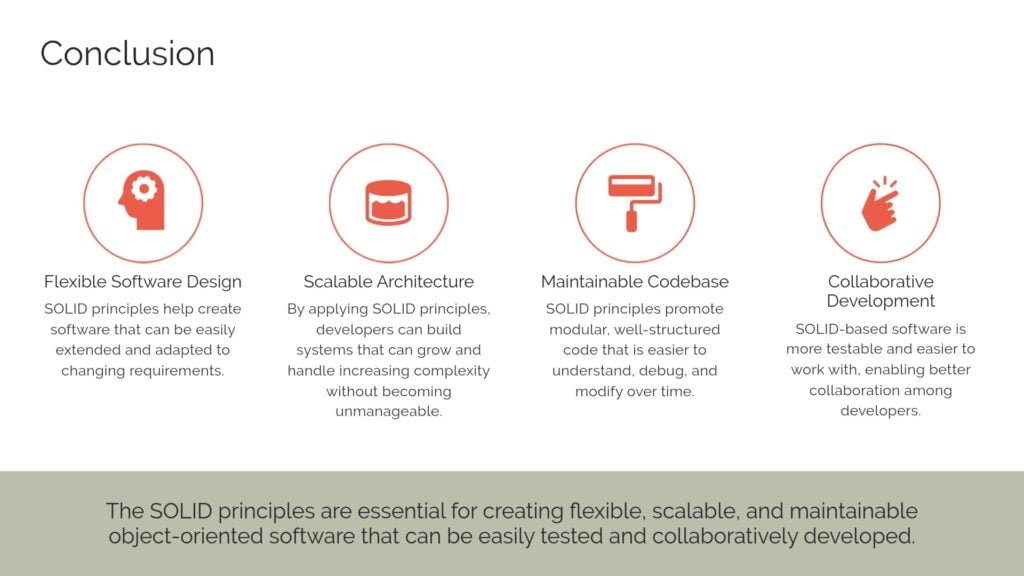
Why Mastering SOLID Matters
By properly applying SOLID, you will:
🚀 Reduce code complexity and improve maintainability.
🚀 Enable faster feature development without breaking existing functionality.
🚀 Improve testability by ensuring modules are decoupled and focused.
🚀 Make it easier for teams to collaborate on large projects.
What’s Next?
Now that you have a solid understanding of SOLID principles, try applying them to your next project! Look for areas where violations might exist and refactor your code to be more modular and scalable.